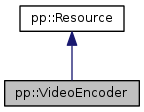
Public Member Functions
VideoEncoder () | |
VideoEncoder (const InstanceHandle &instance) | |
VideoEncoder (const VideoEncoder &other) | |
int32_t | GetSupportedProfiles (const CompletionCallbackWithOutput< std::vector< PP_VideoProfileDescription > > &cc) |
int32_t | Initialize (const PP_VideoFrame_Format &input_format, const Size &input_visible_size, const PP_VideoProfile &output_profile, const uint32_t initial_bitrate, PP_HardwareAcceleration acceleration, const CompletionCallback &cc) |
int32_t | GetFramesRequired () |
int32_t | GetFrameCodedSize (Size *coded_size) |
int32_t | GetVideoFrame (const CompletionCallbackWithOutput< VideoFrame > &cc) |
int32_t | Encode (const VideoFrame &video_frame, bool force_keyframe, const CompletionCallback &cc) |
int32_t | GetBitstreamBuffer (const CompletionCallbackWithOutput< PP_BitstreamBuffer > &cc) |
void | RecycleBitstreamBuffer (const PP_BitstreamBuffer &bitstream_buffer) |
void | RequestEncodingParametersChange (uint32_t bitrate, uint32_t framerate) |
void | Close () |
Detailed Description
Video encoder interface.
Typical usage:
- Call Create() to create a new video encoder resource.
- Call GetSupportedFormats() to determine which codecs and profiles are available.
- Call Initialize() to initialize the encoder for a supported profile.
- Call GetVideoFrame() to get a blank frame and fill it in, or get a video frame from another resource, e.g.
PPB_MediaStreamVideoTrack
. - Call Encode() to push the video frame to the encoder. If an external frame is pushed, wait for completion to recycle the frame.
- Call GetBitstreamBuffer() continuously (waiting for each previous call to complete) to pull encoded pictures from the encoder.
- Call RecycleBitstreamBuffer() after consuming the data in the bitstream buffer.
- To destroy the encoder, the plugin should release all of its references to it. Any pending callbacks will abort before the encoder is destroyed.
Available video codecs vary by platform. All: vp8 (software). ChromeOS, depending on your device: h264 (hardware), vp8 (hardware)
Constructor & Destructor Documentation
Default constructor for creating an is_null() VideoEncoder
object.
pp::VideoEncoder::VideoEncoder | ( | const InstanceHandle & | instance | ) | [explicit] |
A constructor used to create a VideoEncoder
and associate it with the provided Instance
.
- Parameters:
[in] instance The instance with which this resource will be associated.
pp::VideoEncoder::VideoEncoder | ( | const VideoEncoder & | other | ) |
The copy constructor for VideoEncoder
.
- Parameters:
[in] other A reference to a VideoEncoder
.
Member Function Documentation
void pp::VideoEncoder::Close | ( | ) |
Closes the video encoder, and cancels any pending encodes.
Any pending callbacks will still run, reporting PP_ERROR_ABORTED
. It is not valid to call any encoder functions after a call to this method. Note: Destroying the video encoder closes it implicitly, so you are not required to call Close().
int32_t pp::VideoEncoder::Encode | ( | const VideoFrame & | video_frame, |
bool | force_keyframe, | ||
const CompletionCallback & | cc | ||
) |
Encodes a video frame.
- Parameters:
[in] video_frame The VideoFrame
to be encoded.[in] force_keyframe A PP_Bool> specifying whether the encoder should emit a key frame for this video frame.
[in] callback A
CompletionCallback
to be called upon completion. Plugins that passVideoFrame
resources owned by other resources should wait for completion before reusing them.
- Returns:
An int32_t containing an error code from
pp_errors.h
. Returns PP_ERROR_FAILED if Initialize() has not successfully completed.
int32_t pp::VideoEncoder::GetBitstreamBuffer | ( | const CompletionCallbackWithOutput< PP_BitstreamBuffer > & | cc | ) |
Gets the next encoded bitstream buffer from the encoder.
- Parameters:
[out] bitstream_buffer A PP_BitstreamBuffer
containing encoded video data.[in] callback A CompletionCallbackWithOutput
to be called upon completion with the next bitstream buffer. The plugin can call GetBitstreamBuffer from the callback in order to continuously "pull" bitstream buffers from the encoder.
- Returns:
- An int32_t containing an error code from
pp_errors.h
. Returns PP_ERROR_FAILED if Initialize() has not successfully completed. Returns PP_ERROR_INPROGRESS if a prior call to GetBitstreamBuffer() has not completed.
int32_t pp::VideoEncoder::GetFrameCodedSize | ( | Size * | coded_size | ) |
Gets the coded size of the video frames required by the encoder.
Coded size is the logical size of the input frames, in pixels. The encoder may have hardware alignment requirements that make this different from |input_visible_size|, as requested in the call to Initialize().
- Parameters:
[in] coded_size A Size
to hold the coded size.
- Returns:
- An int32_t containing a result code from
pp_errors.h
. Returns PP_ERROR_FAILED if Initialize() has not successfully completed.
int32_t pp::VideoEncoder::GetFramesRequired | ( | ) |
Gets the number of input video frames that the encoder may hold while encoding.
If the plugin is providing the video frames, it should have at least this many available.
- Returns:
- An int32_t containing the number of frames required, or an error code from
pp_errors.h
. Returns PP_ERROR_FAILED if Initialize() has not successfully completed.
int32_t pp::VideoEncoder::GetSupportedProfiles | ( | const CompletionCallbackWithOutput< std::vector< PP_VideoProfileDescription > > & | cc | ) |
Gets an array of supported video encoder profiles.
These can be used to choose a profile before calling Initialize().
- Parameters:
[in] callback A CompletionCallbackWithOutput
to be called upon completion with the PP_VideoProfileDescription structs.
- Returns:
- If >= 0, the number of supported profiles returned, otherwise an error code from
pp_errors.h
.
int32_t pp::VideoEncoder::GetVideoFrame | ( | const CompletionCallbackWithOutput< VideoFrame > & | cc | ) |
Gets a blank video frame which can be filled with video data and passed to the encoder.
- Parameters:
[in] callback A CompletionCallbackWithOutput
to be called upon completion with the blankVideoFrame
resource.
- Returns:
- An int32_t containing an error code from
pp_errors.h
.
int32_t pp::VideoEncoder::Initialize | ( | const PP_VideoFrame_Format & | input_format, |
const Size & | input_visible_size, | ||
const PP_VideoProfile & | output_profile, | ||
const uint32_t | initial_bitrate, | ||
PP_HardwareAcceleration | acceleration, | ||
const CompletionCallback & | cc | ||
) |
Initializes a video encoder resource.
This should be called after GetSupportedProfiles() and before any functions below.
- Parameters:
[in] input_format The PP_VideoFrame_Format
of the frames which will be encoded.[in] input_visible_size A Size
specifying the dimensions of the visible part of the input frames.[in] output_profile A PP_VideoProfile
specifying the codec profile of the encoded output stream.[in] acceleration A PP_HardwareAcceleration
specifying whether to use a hardware accelerated or a software implementation.[in] callback A CompletionCallback
to be called upon completion.
- Returns:
- An int32_t containing an error code from
pp_errors.h
. Returns PP_ERROR_NOTSUPPORTED if video encoding is not available, or the requested codec profile is not supported. Returns PP_ERROR_NOMEMORY if frame and bitstream buffers can't be created.
void pp::VideoEncoder::RecycleBitstreamBuffer | ( | const PP_BitstreamBuffer & | bitstream_buffer | ) |
Recycles a bitstream buffer back to the encoder.
- Parameters:
[in] bitstream_buffer A PP_BitstreamBuffer
that is no longer needed by the plugin.
void pp::VideoEncoder::RequestEncodingParametersChange | ( | uint32_t | bitrate, |
uint32_t | framerate | ||
) |
Requests a change to encoding parameters.
This is only a request, fulfilled on a best-effort basis.
- Parameters:
[in] bitrate The requested new bitrate, in bits per second. [in] framerate The requested new framerate, in frames per second.
The documentation for this class was generated from the following file: